One day, I was playing around and I found this cool site that showed
how to display 2 sides of a rotating cube using OpenGL and Python. I hadn't ever thought that I could
use python and OpenGL but it appears that was the key to easily understanding it all along. Let me explain:
OpenGL is already an advanced and complicated topic. Most people can agree that there isn't an easy way to
learn, you just have to dive right in. I can agree to an extent. Using python, we can use Pygame to create
a window. This makes programming OpenGL a breeze for simple projects because you don't have to understand the
scary OpenGL window creation rules. Thus, my journey had begun.
Simple Triangle: Wed. Nov 20, 2024 | 12:00AM
Although this is a simple triangle, it was really easy to understand. It was only 45 lines of code and it can be compressed to much less. I only used python.
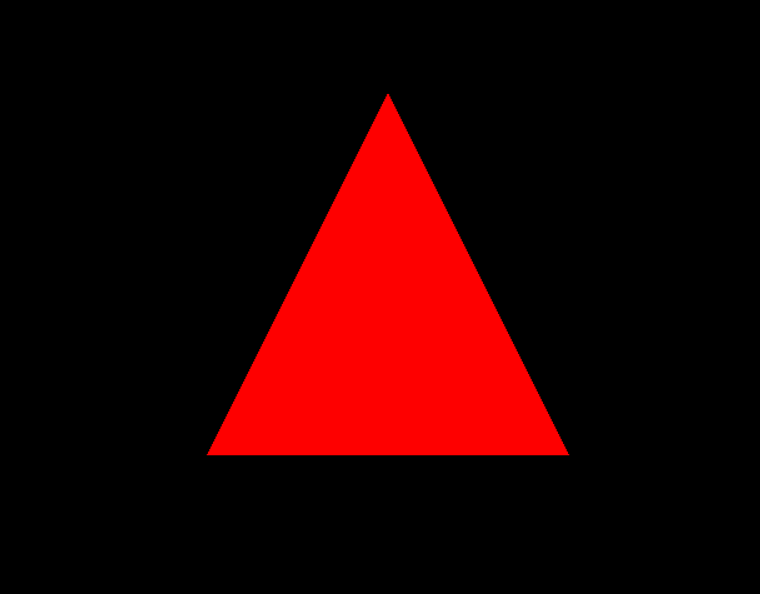
Colorful Triangle: Wed. Nov 20, 2024 | 12:00AM
The colorful Triangle. This was very satisfying. Although I didn't do a whole lot to make this look pretty, it did the color shading itself.
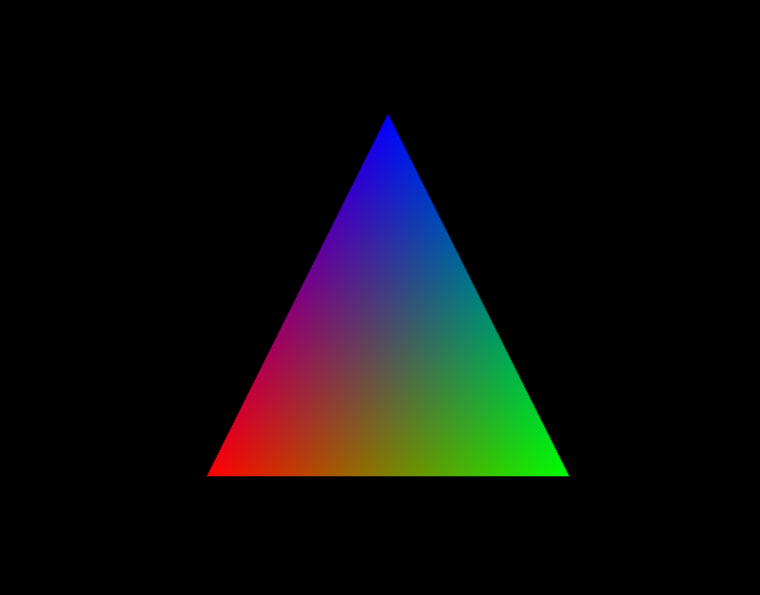
Rotating Cube: Wed. Nov 20, 2024 | 12:00AM
I will admit, this was the very first thing that started this journey. At the time, I really liked this cube, but there was just one problem. You can see that certain faces are being drawn on top of other faces. I was and am currently still inexperienced. I had no clue where to start by fixing this, so I routed to the triangle. I will say, it is a neat effect and I think I can see how this can be used for some transparency effects.
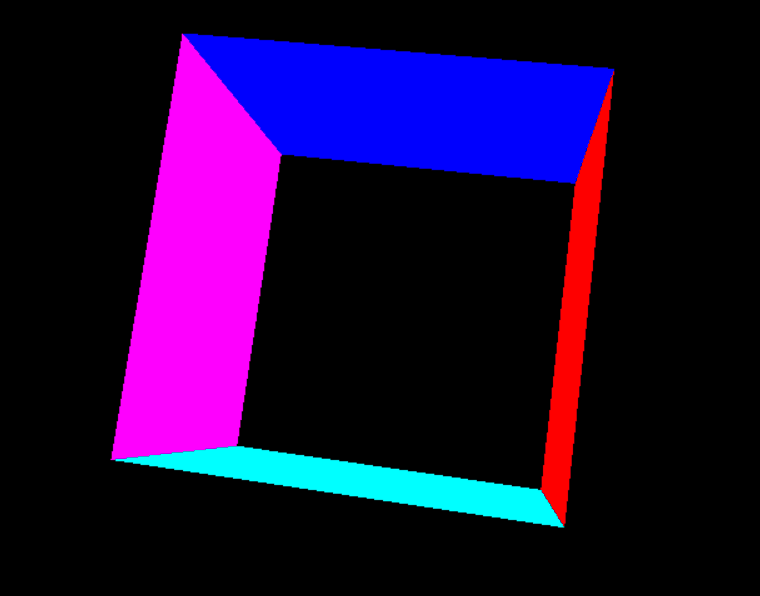
Outlined Cube: Thu. Nov 21, 2024 | 12:00AM
This Cube is cool looking! An article helped me draw this cube. It looks cool and retro; However, it still isn't what I want. I want a 3D cube with faces that you cannot see through. I will still count this as a win! Link To wire Cube
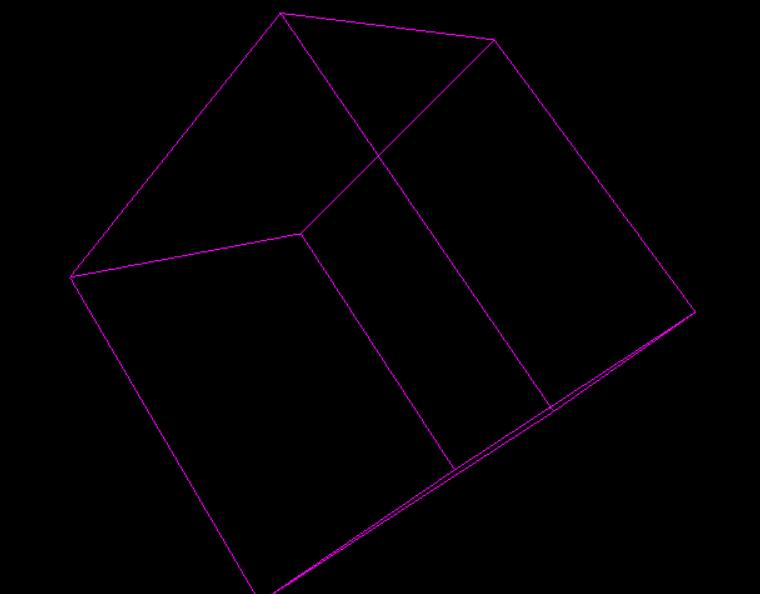
The Perfect Cube: Thu. Nov 21, 2024 | 12:00AM
I've done it!!! Its 9pm and With some research thanks to LearnOpenGl.com I learned about this cool thing called Face Culling. This is the idea that if you draw the face counter-clockwise, you can see it. If you draw it clockwise, then you can't see it. If the cube is rotating then the back side becomes counter-clockwise when it is facing you so you can see it and the front side is now on the back. So now the cube looks like this!
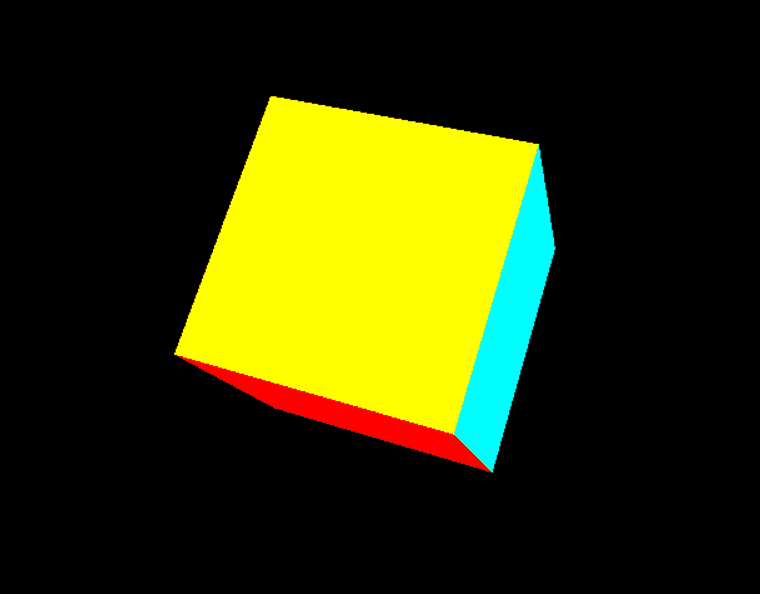
Movement: Fri. Nov 22, 2024 | 12:00AM
What's next after the rotating cube? Many things. I can learn how to import models, draw textures, draw more blocks, make mini games, and many, many.. more. I figured the best way to start was movement. So I made this cube and I made it so that I can move left, right, forward, and backward. Take a look!
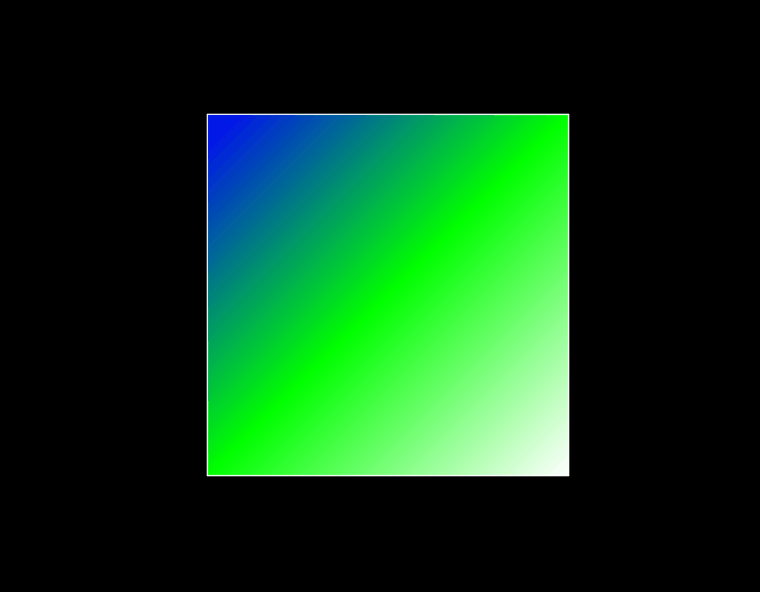
Doom Scene: Sat. Nov 23, 2024 | 12:00AM
Welcome! Today's topic is intense, so get ready for a ride. So this morning, I was inspired by my primitive computer drawings. What is better than a cube? (Not much honestly) A scene! I wanted to draw a scene inspired by the classic Doom games. I will be honest, I don't know how they did it, but I can infer and use my own tactics. Lets break it down. What is in a Doom scene? First, we have walls. This is the main focus today. Next we have a floor, and then sometimes we have a ceiling. I am really only thinking about how to draw the walls. I am going to do this with an array. I am using python so I will be referring to it as a list from now on. What is the first thing we need to do to do? We need to make a 2Dimensional list, I'll go ahead and do that:
[[1, 1, 1, 1, 1, 1],
[1, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 1],
[1, 0, 1, 0, 0, 1],
[1, 0, 5, 0, 0, 1],
[1, 1, 1, 1, 1, 1]]
Lets explain what everything is:
Walls = 1
Air = 0 (This essentially means that nothing is drawn)
Player = 5
The map is laid out from a top down view, you can see that there are walls surrounding the player with one singular wall near the middle.
An important note, graphics are heavy on the GPU/CPU. Cutting how much you are drawing is extremely important. Especially back when doom was made. So I need to draw the least amount of walls possible. I plan to write an algorithm that checks if there is a wall that is touching another wall. If that is the case, then we shouldn't draw the wall.
I didn't fully complete it but I am happy with it. From above it looks broken but if you were on the scene, it would be hard to tell what was broken. I didn't draw the top where the cubes were because the player wouldn't be able to see that high. I also implemented a feature where the walls wouldn't draw if they couldn't be seen due to a wall being connected to it. I was generating the gif below is a render from the 2D list above. If I change any numbers in that list, A wall will be drawn there. I do plan to come back to this later and apply textures and more neat things to this. But for now, another project is calling me!
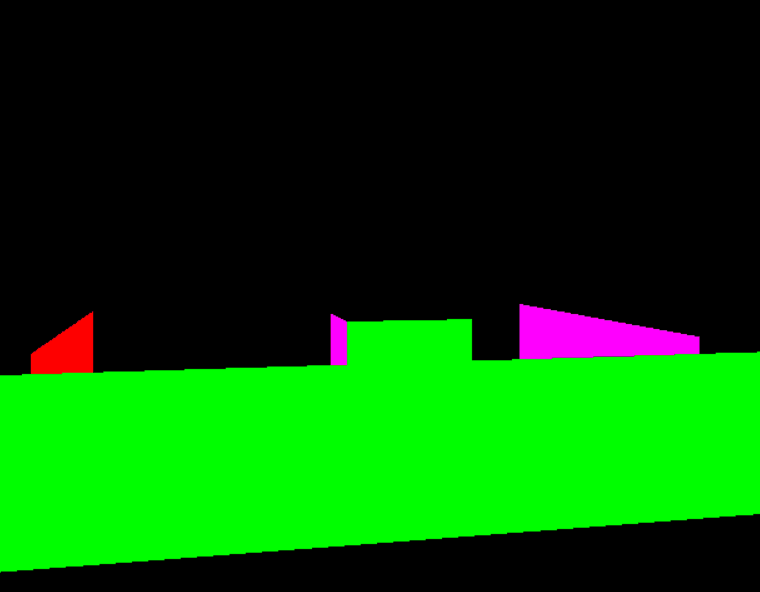
Mini Minecraft Chunk: Sun. Nov 24, 2024 | 12:00AM
I stopped the doom map generation because of this. I wanted to draw a minecraft chunk!!! I actually learned a few things that would've been incredibly helpful for the doom map generator. During this project.
glEnable(GL_DEPTH_TEST)
Depth test is about what it sounds like. If something is further, it can't be seen but it will be drawn. Face Culling helps optimise things but Depth Testing needs to be used when Face Culling doesn't work. For the doom scene, I drew walls based on what direction they were facing. For example, south walls would start drawing in the back and move closer to the player. North walls draw the opposite way. Depth Testing solved this issue. Now for the minecraft Chunk. It is horribly optimised because currently the chunk is 16x16x8 blocks wide, thats 2048 blocks being drawn all at once. Good news is that I have face Culling working properly so 2048 to 4096 faces are being drawn instead of 4096 to 8192 faces. I need to make it so that certain faces do not draw if they are connected to blocks. This shouldn't be difficult as I already did that for the doom map. This should cut down the amount of faces being drawn to only 128 to 256 when being rotated like we currently see. Once I fix this, the chunk can be full sized rather than just 8 blocks deep.
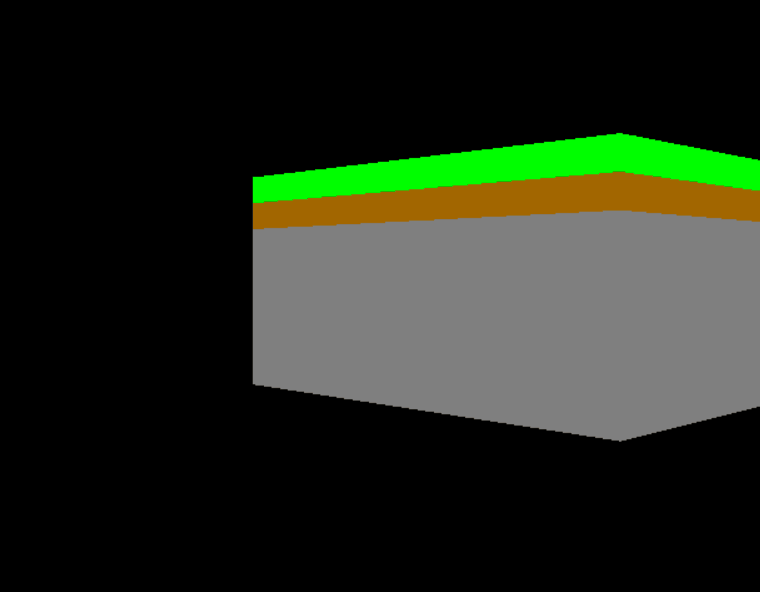